Deck 10: Classes and Data Abstraction
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
فتح الحزمة
قم بالتسجيل لفتح البطاقات في هذه المجموعة!
Unlock Deck
Unlock Deck
1/50
العب
ملء الشاشة (f)
Deck 10: Classes and Data Abstraction
1
As parameters to a function,class objects can be passed by reference only.
False
2
A class is an example of a structured data type.
True
3
If a member of a class is ____,you cannot access it outside the class.
A) public
B) automatic
C) private
D) static
A) public
B) automatic
C) private
D) static
C
4
Given the declaration
class myClass
{
public:
void print(); //Output the value of x;
MyClass();
private:
int x;
};
myClass myObject;
The following statement is legal.
myObject.x = 10;
class myClass
{
public:
void print(); //Output the value of x;
MyClass();
private:
int x;
};
myClass myObject;
The following statement is legal.
myObject.x = 10;
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
5
If the heading of a member function of a class ends with the word const,then the function member cannot modify the private member variables,but it can modify the public member variables.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
6
The public members of a class must be declared before the private members.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
7
Figure 1:
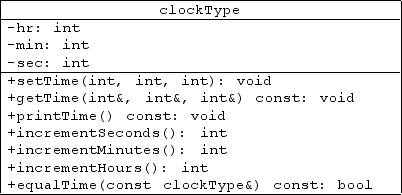
The word ____ at the end of the member functions in the accompanying class clockType in Figure 1 specifies that these functions cannot modify the member variables of a clockType object.
A) static
B) const
C) automatic
D) private
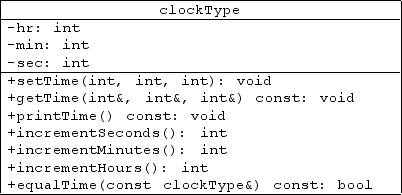
The word ____ at the end of the member functions in the accompanying class clockType in Figure 1 specifies that these functions cannot modify the member variables of a clockType object.
A) static
B) const
C) automatic
D) private
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
8
Figure 1:
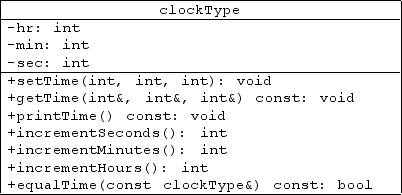
Consider the UML class diagram shown in the accompanying figure.According to the UML class diagram,how many private members are in the class?
A) none
B) zero
C) two
D) three
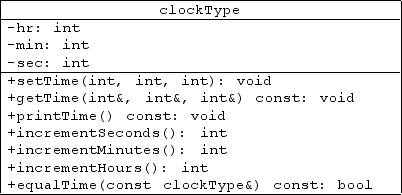
Consider the UML class diagram shown in the accompanying figure.According to the UML class diagram,how many private members are in the class?
A) none
B) zero
C) two
D) three
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
9
A ____ sign in front of a member name on a UML diagram indicates that this member is a protected member.
A) +
B) -
C) #
D) $
A) +
B) -
C) #
D) $
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
10
Figure 2:
class rectangleType
{
public:
void setLengthWidth(double x, double y);
//Postcondition: length = x; width = y;
void print() const;
//Output length and width;
double area();
//Calculate and return the area of the rectangle;
double perimeter();
//Calculate and return the parameter;
rectangleType();
//Postcondition: length = 0; width = 0;
rectangleType(double x, double y);
//Postcondition: length = x; width = y;
private:
double length;
double width;
};
Consider the accompanying class definition in Figure 2.Which of the following variable declarations is correct?
A) rectangle rectangleType;
B) class rectangleType rectangle;
C) rectangleType rectangle;
D) rectangle rectangleType.area;
class rectangleType
{
public:
void setLengthWidth(double x, double y);
//Postcondition: length = x; width = y;
void print() const;
//Output length and width;
double area();
//Calculate and return the area of the rectangle;
double perimeter();
//Calculate and return the parameter;
rectangleType();
//Postcondition: length = 0; width = 0;
rectangleType(double x, double y);
//Postcondition: length = x; width = y;
private:
double length;
double width;
};
Consider the accompanying class definition in Figure 2.Which of the following variable declarations is correct?
A) rectangle rectangleType;
B) class rectangleType rectangle;
C) rectangleType rectangle;
D) rectangle rectangleType.area;
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
11
In C++,class is a reserved word and it defines only a data type.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
12
If an object is created in a user program,then the object can access both the public and private members of the class.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
13
In C++ terminology,a class object is the same as a class instance.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
14
A ____ sign in front of a member name on a UML diagram indicates that this member is a public member.
A) +
B) -
C) #
D) $
A) +
B) -
C) #
D) $
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
15
Which of the following class definitions is correct in C++?
A) class studentType
{
Public:
Void setData(string, double, int);
Private:
String name;
};
B) class studentType
{
Public:
Void setData(string, double, int);
Void print() const;
Private:
String name;
Double gpa;
}
C) class studentType
{
Public void setData(string, double, int);
Private string name;
};
D) studentType class
{
Public: void setData(string, double, int);
Private: string name;
};
A) class studentType
{
Public:
Void setData(string, double, int);
Private:
String name;
};
B) class studentType
{
Public:
Void setData(string, double, int);
Void print() const;
Private:
String name;
Double gpa;
}
C) class studentType
{
Public void setData(string, double, int);
Private string name;
};
D) studentType class
{
Public: void setData(string, double, int);
Private: string name;
};
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
16
If an object is declared in the definition of a member function of the class,then the object can access both the public and private members of the class.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
17
Figure 1:
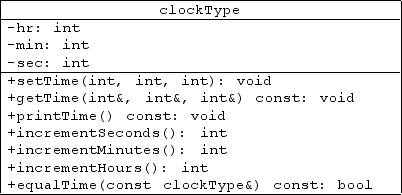
Consider the UML class diagram shown in the accompanying figure.Which of the following is the name of the class?
A) clock
B) clockType
C) Type
D) +clockType
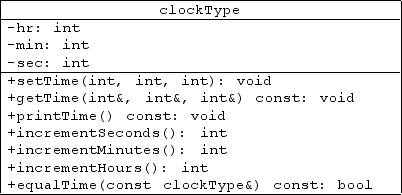
Consider the UML class diagram shown in the accompanying figure.Which of the following is the name of the class?
A) clock
B) clockType
C) Type
D) +clockType
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
18
The components of a class are called the ____ of the class.
A) elements
B) members
C) objects
D) properties
A) elements
B) members
C) objects
D) properties
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
19
You can use arithmetic operators to perform arithmetic operations on class objects.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
20
A class and its members can be described graphically using a notation known as the ____ notation.
A) OON
B) OOD
C) UML
D) OOP
A) OON
B) OOD
C) UML
D) OOP
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
21
A destructor has the character ____,followed by the name of the class.
A) .
B) ::
C) #
D) ~
A) .
B) ::
C) #
D) ~
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
22
In C++,you can pass a variable by reference and still prevent the function from changing its value by using the keyword ____ in the formal parameter declaration.
A) automatic
B) private
C) static
D) const
A) automatic
B) private
C) static
D) const
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
23
If a function of a class is static,it is declared in the class definition using the keyword static in its ____.
A) return type
B) parameters
C) heading
D) main function
A) return type
B) parameters
C) heading
D) main function
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
24
A program or software that uses and manipulates the objects of a class is called a(n)____________________ of that class.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
25
If a class object is passed by ____________________,the contents of the member variables of the actual parameter are copied into the corresponding member variables of the formal parameter.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
26
In C++,the scope resolution operator is ____.
A) :
B) ::
C) $
D) .
A) :
B) ::
C) $
D) .
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
27
Figure 2:
class rectangleType
{
public:
void setLengthWidth(double x, double y);
//Postcondition: length = x; width = y;
void print() const;
//Output length and width;
double area();
//Calculate and return the area of the rectangle;
double perimeter();
//Calculate and return the parameter;
rectangleType();
//Postcondition: length = 0; width = 0;
rectangleType(double x, double y);
//Postcondition: length = x; width = y;
private:
double length;
double width;
};
Consider the accompanying class definition,and the object declaration: rectangleType bigRect(14,10);
Which of the following statements is correct?
A) bigRect.setLengthWidth();
B) bigRect.setLengthWidth(3.0, 2.0);
C) bigRect.length = 2.0;
D) bigRect.length = bigRect.width;
class rectangleType
{
public:
void setLengthWidth(double x, double y);
//Postcondition: length = x; width = y;
void print() const;
//Output length and width;
double area();
//Calculate and return the area of the rectangle;
double perimeter();
//Calculate and return the parameter;
rectangleType();
//Postcondition: length = 0; width = 0;
rectangleType(double x, double y);
//Postcondition: length = x; width = y;
private:
double length;
double width;
};
Consider the accompanying class definition,and the object declaration: rectangleType bigRect(14,10);
Which of the following statements is correct?
A) bigRect.setLengthWidth();
B) bigRect.setLengthWidth(3.0, 2.0);
C) bigRect.length = 2.0;
D) bigRect.length = bigRect.width;
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
28
By default,all members of a class are ____________________.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
29
Figure 2:
class rectangleType
{
public:
void setLengthWidth(double x, double y);
//Postcondition: length = x; width = y;
void print() const;
//Output length and width;
double area();
//Calculate and return the area of the rectangle;
double perimeter();
//Calculate and return the parameter;
rectangleType();
//Postcondition: length = 0; width = 0;
rectangleType(double x, double y);
//Postcondition: length = x; width = y;
private:
double length;
double width;
};
Consider the accompanying class definition,and the declaration: rectangleType bigRect;
Which of the following statements is correct?
A) rectangleType.print();
B) rectangl
C) bigRect.print();
D) bigRect::print();
class rectangleType
{
public:
void setLengthWidth(double x, double y);
//Postcondition: length = x; width = y;
void print() const;
//Output length and width;
double area();
//Calculate and return the area of the rectangle;
double perimeter();
//Calculate and return the parameter;
rectangleType();
//Postcondition: length = 0; width = 0;
rectangleType(double x, double y);
//Postcondition: length = x; width = y;
private:
double length;
double width;
};
Consider the accompanying class definition,and the declaration: rectangleType bigRect;
Which of the following statements is correct?
A) rectangleType.print();
B) rectangl
C) bigRect.print();
D) bigRect::print();
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
30
A class object can be ____.That is,it is created each time the control reaches its declaration,and destroyed when the control exits the surrounding block.
A) static
B) automatic
C) local
D) public
A) static
B) automatic
C) local
D) public
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
31
What does ADT stand for?
A) abstract definition type
B) asynchronous data transfer
C) abstract data type
D) alternative definition type
A) abstract definition type
B) asynchronous data transfer
C) abstract data type
D) alternative definition type
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
32
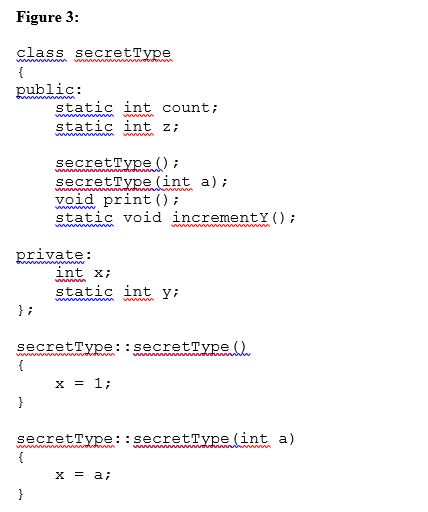
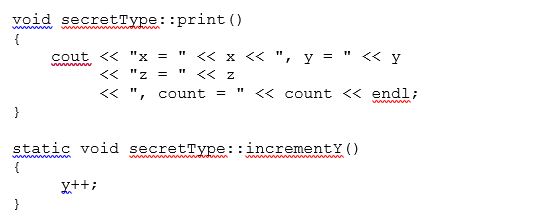
How many destructors can a class have?
A) no explicit destructors
B) one
C) two
D) any number
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
33
A member function of a class that only accesses the value(s)of the data member(s)is called a(n)____ function.
A) accessor
B) mutator
C) constructor
D) destructor
A) accessor
B) mutator
C) constructor
D) destructor
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
34
Non-static member variables of a class are called the ____________________ variables of the class.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
35
In C++,the ____ is an operator called the member access operator.
A) .
B) ,
C) ::
D) #
A) .
B) ,
C) ::
D) #
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
36
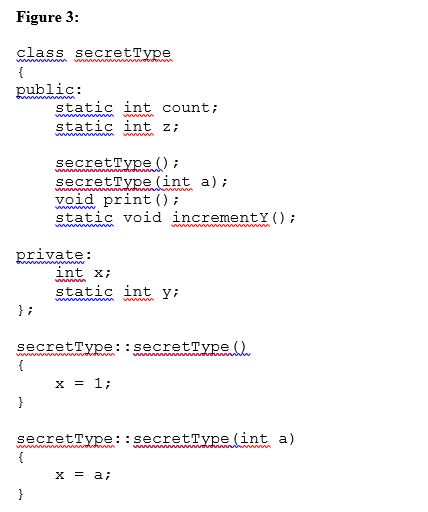
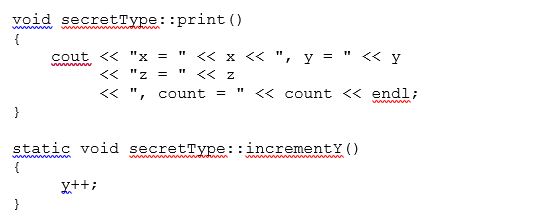
Consider the accompanying class and member functions definitions in Figure 3.How many constructors are present in the class definition above?
A) none
B) one
C) two
D) three
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
37
To guarantee that the member variables of a class are initialized,you use ____.
A) accessors
B) mutators
C) constructors
D) destructor
A) accessors
B) mutators
C) constructors
D) destructor
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
38
A class object can be ____.That is,it can be created once,when the control reaches its declaration,and destroyed when the program terminates.
A) static
B) automatic
C) local
D) public
A) static
B) automatic
C) local
D) public
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
39
A(n)____________________ function of a class changes the values of the member variable(s)of the class.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
40
Which of the following is true about classes and structs?
A) By default, all members of a struct are public and all members of a class are private.
B) A struct variable is passed by value only, and a class variable is passed by reference only.
C) An assignment operator is allowed on class variables, but not on struct variables.
D) You cannot use the member access specifier private in a struct.
A) By default, all members of a struct are public and all members of a class are private.
B) A struct variable is passed by value only, and a class variable is passed by reference only.
C) An assignment operator is allowed on class variables, but not on struct variables.
D) You cannot use the member access specifier private in a struct.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
41
A constructor with no parameters is called the ____________________ constructor.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
42
A(n)____________________ statement is required by any program that uses a header class file,as well as by the implementation file that defines the operations for that class.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
43
Object code is produced from a(n)____________________.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
44
The header file is also known as the ____________________.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
45
Classes were specifically designed in C++ to handle ____________________.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
46
A(n)____________________ contains the definitions of the functions to implement the operations of an object.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
47
A(n)____________________ is a statement specifying what is true after the function call is completed.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
48
A C++ implementation file has the extension ____________________.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
49
A(n)____________________ is a statement specifying the condition(s)that must be true before the function is called.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck
50
To create the object code file for any source code file,the command line option ____________________ is used on the system command line.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 50 في هذه المجموعة.
فتح الحزمة
k this deck